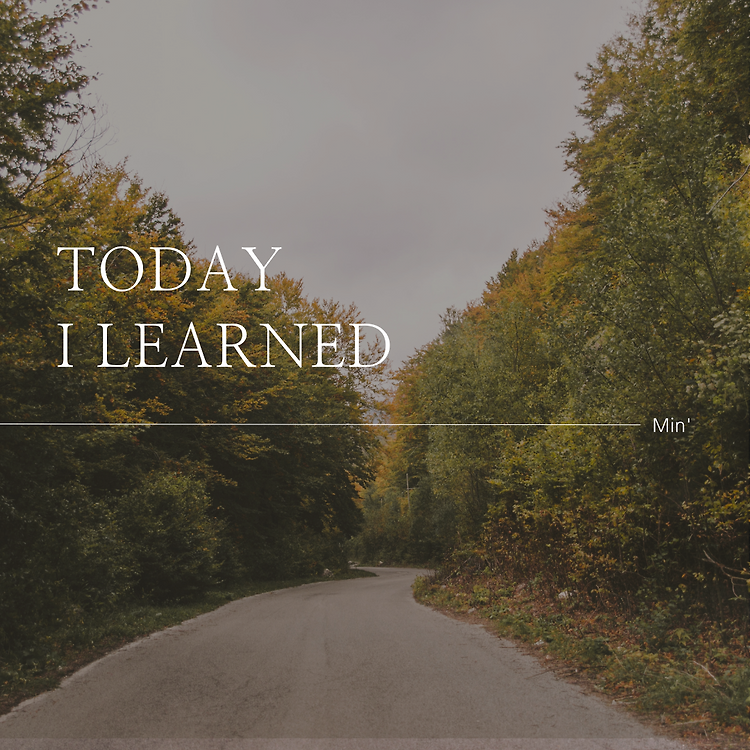
- useState를 활용한 useTabs
웹사이트에 메뉴 또는 무엇이든 간에 tab을 사용하기 매우 쉽게 만들어주는 것
1. content라는 상수를 선언해 배열 담아주기
2. map을 이용하여 component에 배열을 렌더링해줌.
map을 돌린 다음, 안에 button을 생성해 object 안의 tab을 담아줌.
Section1 button을 클릭했을 때 section1 content만, Section2 button을 클릭했을 때 section2 content만
보이게끔 하는 것이 목표.
3. useTabs hook 생성
3-1. initialTab(초기 탭)과 allTabs(모든 탭)을 매개변수로 담아줌
3-2. App component로 가 tabs라는 상수를 선언해 useTabs(0, content)을 담아줌
3-3. 현재 선택한 탭의 content를 얻고 싶음 => 현재 선택한 content의 index를 얻고 싶다는 뜻
useTabs function 내부에 currentItem을 return해줌
allTabs 매개변수에 content를 담아주고 currentItem은 allTabs[currentIndex]를 담아줌
3-4. array를 검증하는 3항연산자 사용 (content는 배열 형태)
만약 not(allTabs 상수가 true) or not(allTabs 상수가 array가 true)라면 return(none)함.
allTabs에 들어갈 값이 존재하는지와 array인지 확인할 수 있음
currentItem.content가 화면에 보이게끔 해줌.
이제 선택한 탭의 content만 화면에 보이게 될 거임.
3-5. useTabs function 내부에 currentItem과 changeItem을 return해줌.
currentItem은 allTabs[currentIndex]를, changeItem은 setCurrentIndex를 담아줌.
3-6. return으로 잡았던 { currentItem, changeItem }을 상수로 잡고 이를 useTabs(0, content)와 동일시함.
즉, 0은 initialTab인 최초값이고 content는 allTabs.
3-7. content로 map을 돌리면서 매개변수로 section과 index를 받음
button을 onClick하면 changeItem의 index를 받게 됨.
import { useState } from "react";
import "./styles.css";
const content = [
{
tab: "Section 1",
content: "I'm the content of the Section 1"
},
{
tab: "Section 2",
content: "I'm the content of the Section 2"
}
];
const useTabs = (initialTab, allTabs) => {
if (!allTabs || !Array.isArray(allTabs)) {
return;
}
const [currentIndex, setCurrentIndex] = useState(initialTab);
return {
currentItem: allTabs[currentIndex],
changeItem: setCurrentIndex
};
};
export default function App() {
const { currentItem, changeItem } = useTabs(0, content);
return (
<div className="App">
{content.map((section, index) => {
return (
<button key={index} onClick={() => changeItem(index)}>
{section.tab}
</button>
);
})}
<div>{currentItem.content}</div>
</div>
);
}
'(심층)리액트' 카테고리의 다른 글
트위터 클론코딩(with firebase) - 02 (0) | 2023.02.01 |
---|---|
트위터 클론코딩(with firebase) - 01 (6) | 2023.02.01 |
React Hooks - 02 / useState를 활용한 useInput (0) | 2023.01.31 |
React Hooks - 01 (1) | 2023.01.26 |
리액트 심화2 - Axios (0) | 2023.01.19 |
github : https://github.com/dnjfht
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!